There are number of operation which we can perform overs the date in objective-c like adding days to date, adding hours to date, date with days before now etc, Here is one cool utility kit available on GitHub which does all these operations for you, all you have to do is just add this to your project and you are ready to go with it.
Here are various operations, which you can perform with it, operation names are self-explanatory.
You can download the library from this link https://github.com/erica/NSDate-Extensions/blob/master/NSDate-Utilities.h
or you can download the project which i made using this library from my GitHub from this link https://github.com/malkitsingh/nsdateutilityapp
How to use it in Xcode:-
1. Open Xcode, and create a single view application, though we will not be using anything of iPhone screen to display (I’ll demonstrate this library using NSLog).
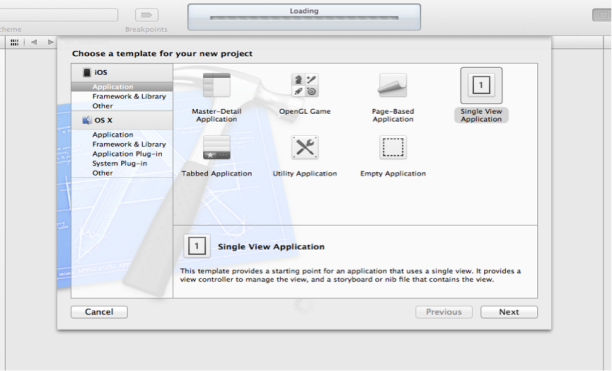
2. Drag and drop NSDate-Utilities.h and NSDate-Utilities.m file into your project.
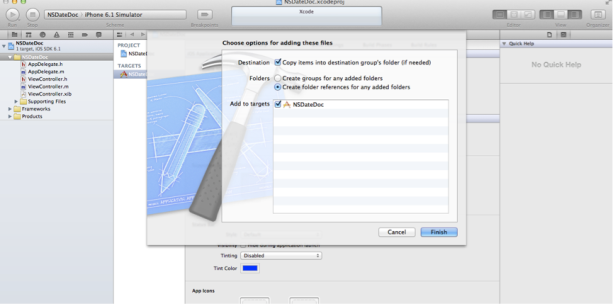
Let’s begin with implementing this extension class.
• First of all import the NSDate-Extension library into your implementation file using this line of code
#import "NSDate-Utilities.h"
Methods in this class are grouped as their functionality.
First group is for Relative dates from the current date
1. dateTomorrow:- this function returns the tomorrows date and doesn’t expects any argument.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"MMM dd, yyyy"];
NSDate *date = [NSDate date];
NSDate *dateTomorrow = [NSDate dateTomorrow];
NSString *formattedDateString = [dateFormatter stringFromDate:date];
NSString *formattedDateStringTomorrow = [dateFormatter stringFromDate:dateTomorrow];
NSLog(@"Todays Date is %@",formattedDateString );
NSLog(@"Tomorrows Date is %@",formattedDateStringTomorrow );
}
2. dateYesterday:– this returns yesterdays as its name suggests.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"MMM dd, yyyy"];
NSDate *date = [NSDate date];
NSDate *dateYest = [NSDate dateYesterday];
NSString *formattedDateString = [dateFormatter stringFromDate:date];
NSString *formattedDateStringyest = [dateFormatter stringFromDate:dateYest];
NSLog(@"Todays Date is %@",formattedDateString );
NSLog(@"Yesterdays Date was %@",formattedDateStringyest );
}
3. + (NSDate *) dateWithDaysFromNow:- (NSInteger) days;- This function returns date by adding no. of days in date of today.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"MMM dd, yyyy"];
NSDate *date = [NSDate date];
NSDate *dateAfternDays = [NSDate dateWithDaysFromNow:2];
NSString *formattedDateString = [dateFormatter stringFromDate:date];
NSString *formattedDateAfternDays = [dateFormatter stringFromDate:dateAfternDays];
NSLog(@"Todays Date is %@",formattedDateString );
NSLog(@"Date After 2 days will be%@",formattedDateAfternDays );
}
4. + (NSDate *) dateWithDaysBeforeNow:-(NSInteger) days; This function does reverse of datewithdaysfromnow, it provides date by subtracting given days from it.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"MMM dd, yyyy"];
NSDate *date = [NSDate date];
NSDate *dateBeforenDays = [NSDate dateWithDaysBeforeNow:2];
NSString *formattedDateString = [dateFormatter stringFromDate:date];
NSString *formattedBeforenDays = [dateFormatter stringFromDate:dateBeforenDays];
NSLog(@"Todays Date is %@",formattedDateString );
NSLog(@"Date Before 2 days was %@",formattedBeforenDays );
}
5. dateWithHoursFromNow :- This function returns us a date by adding no. of hours which we specify.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"MMM dd, yyyy"];
NSDate *date = [NSDate date];
NSDate *dateAfternHoursFromNow = [NSDate dateWithHoursFromNow:20];
NSString *formattedDateString = [dateFormatter stringFromDate:date];
NSString *formattedAfternHoursFromNow = [dateFormatter stringFromDate:dateAfternHoursFromNow];
NSLog(@"Todays Date is %@",formattedDateString );
NSLog(@"Date After 20 hours from now will %@",formattedAfternHoursFromNow );
}
6. + (NSDate *) dateWithHoursBeforeNow: (NSInteger) dHours;- This functions returns date by subtracting given no. of hours from todays date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"MMM dd, yyyy"];
NSDate *date = [NSDate date];
NSDate *dateBeforenHoursFromNow = [NSDate dateWithHoursBeforeNow:20];
NSString *formattedDateString = [dateFormatter stringFromDate:date];
NSString *formattedBeforenHoursFromNow = [dateFormatter stringFromDate:dateBeforenHoursFromNow];
NSLog(@"Todays Date is %@",formattedDateString );
NSLog(@"Date Before 20 hours from now was %@",formattedBeforenHoursFromNow );
}
7. dateWithMinutesFromNow :- as its name suggests, this function returns a date by adding given no. of minutes to it.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"MMM dd, yyyy"];
NSDate *date = [NSDate date];
NSDate *dateAfternMinutesFromNow = [NSDate dateWithMinutesFromNow:5000];
NSString *formattedDateString = [dateFormatter stringFromDate:date];
NSString *formattedAfternMinutesFromNow = [dateFormatter stringFromDate:dateAfternMinutesFromNow];
NSLog(@"Todays Date is %@",formattedDateString );
NSLog(@"Date After 5000 minutess from now will be %@",formattedAfternMinutesFromNow );
}
8. dateWithMinutesBeforeNow:– This functions returns a date from now by subtracting given no. of minutes from it.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"MMM dd, yyyy"];
NSDate *date = [NSDate date];
NSDate *dateBeforenMinutesFromNow = [NSDate dateWithMinutesFromNow:5000];
NSString *formattedDateString = [dateFormatter stringFromDate:date];
NSString *formattedBeforenMinutesFromNow = [dateFormatter stringFromDate:dateBeforenMinutesFromNow];
NSLog(@"Todays Date is %@",formattedDateString );
NSLog(@"Date Before 5000 minutess from now was %@",formattedBeforenMinutesFromNow );
}
8. dateWithMinutesBeforeNow:– This functions returns a date from now by subtracting given no. of minutes from it.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"MMM dd, yyyy"];
NSDate *date = [NSDate date];
NSDate *dateBeforenMinutesFromNow = [NSDate dateWithMinutesFromNow:5000];
NSString *formattedDateString = [dateFormatter stringFromDate:date];
NSString *formattedBeforenMinutesFromNow = [dateFormatter stringFromDate:dateBeforenMinutesFromNow];
NSLog(@"Todays Date is %@",formattedDateString );
NSLog(@"Date Before 5000 minutess from now was %@",formattedBeforenMinutesFromNow );
}
Second group of function is for Comparing Dates:-
Almost every single function of this group returns Boolean result i.e. either true or false.
Let’s explore functions of this group.
1. isEqualToDateIgnoringTime: – this method returns us true or false by comparing two given dates.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date = [NSDate date];
NSDate *dateBeforenMinutesFromNow = [NSDate dateWithMinutesFromNow:5000];
if([date isEqualToDateIgnoringTime:dateBeforenMinutesFromNow])
NSLog(@"Two dates are equal");
else
NSLog(@"Two Dates are not equal");
}
2. – (BOOL) isTomorrow; – This function returns true or false based on result of is given date is tomorrows date or not.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date = [NSDate date];
if([date isTomorrow])
NSLog(@"yes its tomorrow");
else
NSLog(@"no its not tomorrow");
}
3. – (BOOL) isYesterday;- This function returns a result and confirms if given date is yesterday’s date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date = [NSDate date];
if([date isYesterday])
NSLog(@"yes its yesterdays date");
else
NSLog(@"no its not yesterdays date");
}
4. – (BOOL) isToday; – This function returns true if given date is todays date else returns false.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date = [NSDate date];
if([date isToday])
NSLog(@"yes its todays date");
else
NSLog(@"no its not todays date");
}
5. – (BOOL) isSameWeekAsDate: (NSDate *) aDate;- This function compares two given dates and checks if both of these dates are of same week, if yes then it returns true else returns false.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date = [NSDate date];
NSDate *dateBeforenMinutesFromNow = [NSDate dateWithMinutesFromNow:5000];
if([date isSameWeekAsDate:dateBeforenMinutesFromNow])
NSLog(@"yes its same week as given date");
else
NSLog(@"no its not same week as given date");
}
6. – (BOOL) isThisWeek;- This function checks if the given date is from same week of system.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date = [NSDate date];
if([date isThisWeek])
NSLog(@"yes given date is from same week");
else
NSLog(@"no given date is not from same week");
}
7. – (BOOL) isNextWeek; – This function checks, if given date is from next week or not.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date = [NSDate date];
if([date isNextWeek])
NSLog(@"yes given date is from next week");
else
NSLog(@"no given date is not from next week");
}
8. – (BOOL) isLastWeek; – This function checks if given date is from last week of the month.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date = [NSDate date];
if([date isLastWeek])
NSLog(@"yes given date is from last weak of month");
else
NSLog(@"no given date is not from last weak of month");
}
9. – (BOOL) isSameMonthAsDate: (NSDate *) aDate;- This function checks , if the given date is from same month of date with which it is being compared.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date = [NSDate date];
NSDate *dateBeforenMinutesFromNow = [NSDate dateWithMinutesFromNow:5000];
if([date isSameMonthAsDate:dateBeforenMinutesFromNow])
NSLog(@"yes given date is from same month");
else
NSLog(@"no given date is not from same month");
}
DATE ROLES GROUP
1. isTypicallyWorkday: – This function returns us a true if the given date is a working day of week (it assumes there are 7 days in week, and on Saturday and Sunday its not a working day.)
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date= [NSDate date];
if([date isTypicallyWorkday])
NSLog(@"workday");
else
NSLog(@"holiday");
}
2. isTypicallyWeekend:- This function returns us true if given date falls in weekend and otherwise it gives us false.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDate *date= [NSDate date];
if([date isTypicallyWeekend])
NSLog(@"hurrey, its weekend");
else
NSLog(@"no its not weekend");
}
Another group comes after roles group is adjusting dates, functions of this group adds or subtracts days, hours and minutes from the given date.
1. – (NSDate *) dateByAddingDays: (NSInteger) dDays:- This function gives us date by adding given no. of days into date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *formatter = [[NSDateFormatter alloc] init];
[ formatter setDateStyle:NSDateFormatterMediumStyle];
//get the date today
NSString *dateToday = [formatter stringFromDate:[[NSDate date] dateByAddingDays:2]];
NSLog(@"Date by adding 2 days in todays date is %@",dateToday);
}
2. – (NSDate *) dateBySubtractingDays: (NSInteger) dDays; this function returns us a date by subtracting given no. of days from the date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *formatter = [[NSDateFormatter alloc] init];
[formatter setDateStyle:NSDateFormatterMediumStyle];
//get the date today
NSString *dateToday = [formatter stringFromDate:[[NSDate date] dateBySubtractingDays:2]];
NSLog(@"Date by subtacting 2 days from todays date was %@",dateToday);
}
3. – (NSDate *) dateByAddingHours: (NSInteger) dHours; this function returns us a date by adding a given number of hours in it.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *date = [[NSDate date] dateByAddingHours:100];
NSString *theDate = [dateFormat stringFromDate:date];
NSLog(@"date by adding 100 hours in todays date is %@",theDate);
}
4. – (NSDate *) dateBySubtractingHours: (NSInteger) dHours;
This function returns us a date by subtracting given no. of hours from the date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *date = [[NSDate date] dateBySubtractingHours:100];
NSString *theDate = [dateFormat stringFromDate:date];
NSLog(@"date by subtracting 100 hours from todays date was %@",theDate);
}
5. (NSDate *) dateByAddingMinutes: (NSInteger) dMinutes;
This function gives us a date by adding given no. of minutes into it.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *date = [[NSDate date] dateByAddingMinutes:10000];
NSString *theDate = [dateFormat stringFromDate:date];
NSLog(@"date by adding 10000 minutes in todays date is %@",theDate);
}
6. – (NSDate *) dateBySubtractingMinutes: (NSInteger) dMinutes;- This function returns us a date by subtracting given no. of minutes from the date we specify.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *date = [[NSDate date] dateBySubtractingMinutes:10000];
NSString *theDate = [dateFormat stringFromDate:date];
NSLog(@"date by subtracting 10000 minutes from todays date was %@",theDate);
}
7. – (NSDate *) dateAtStartOfDay; this function returns us the date which was there at the start of the given date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *date = [[NSDate date] dateAtStartOfDay];
NSString *theDate = [dateFormat stringFromDate:date];
NSLog(@"date at start of the todays date was %@",theDate);
}
Another group which comes after adjusting dates group is retrieving interval group, all the functions in this group returns us the integer values which can be minutes, hours, days before or after from the given date.
1. – (NSInteger) minutesAfterDate: (NSDate *) aDate:- This function gives us the no. of minutes which are passed after the given date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *datePrevious = [[NSDate date] dateBySubtractingMinutes:1000];
NSDate *dateToday = [NSDate date];
NSLog(@"Minutes spent after subtracting 1000 minutes from todays date are %d",[dateToday minutesAfterDate:datePrevious]);
}
2. – (NSInteger) minutesBeforeDate: (NSDate *) aDate:- This function returns us the minutes remaining before the given date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *dateFuture = [[NSDate date] dateByAddingMinutes:1000];
NSDate *dateToday = [NSDate date];
NSLog(@"Minutes left before date are %d",[dateToday minutesBeforeDate:dateFuture]);
}
3. – (NSInteger) hoursAfterDate: (NSDate *) aDate:- This function returns us no. of hours spent after the date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *dateFuture = [[NSDate date] dateBySubtractingHours:100];
NSDate *dateToday = [NSDate date];
NSLog(@"Hours after date are %d",[dateToday hoursAfterDate:dateFuture]);
}
4. – (NSInteger) hoursBeforeDate: (NSDate *) aDate:- This function return us the no. of hours remaining in given date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *dateFuture = [[NSDate date] dateByAddingHours:100];
NSDate *dateToday = [NSDate date];
NSLog(@"Hours left before date are %d",[dateToday hoursBeforeDate:dateFuture]);
}
5. – (NSInteger) daysAfterDate: (NSDate *) aDate;-This function returns us no. of days spent after a given date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *dateFuture = [[NSDate date] dateBySubtractingDays:3];
NSDate *dateToday = [NSDate date];
NSLog(@"Days after date are %d",[dateToday daysAfterDate:dateFuture]);
}
6. – (NSInteger) daysBeforeDate: (NSDate *) aDate; this function return us no. of days remaining in given date from the reference date.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *dateFuture = [[NSDate date] dateByAddingDays:3];
NSDate *dateToday = [NSDate date];
NSLog(@"Days left before date are %d",[dateToday daysBeforeDate:dateFuture]);
}
7. – (NSInteger)distanceInDaysToDate:(NSDate *)anotherDate:- This function returns us, distance between two dates in days.
- (void)viewDidLoad
{
[super viewDidLoad];
NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init];
[dateFormat setDateFormat:@"yyyy-MM-dd"];
NSDate *dateFuture = [[NSDate date] dateByAddingDays:30];
NSDate *dateToday = [NSDate date]
NSLog(@"Distance in days to date is %d days",[dateToday distanceInDaysToDate:dateFuture]);
}
And lot more like above mentioned methods, for any further details and updated on the library please visit the GitHub address which I mentioned above in this post.
For more updates and such new code tricks visit codeios.net
Happy coding!